Sharp Waves - Maths317
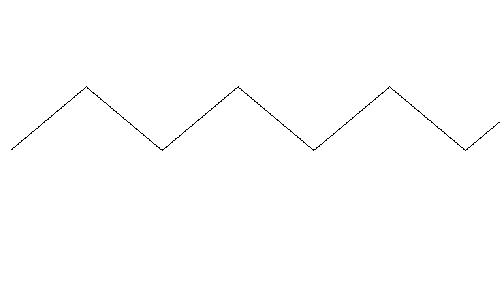
We would be using the line formula in the lines chapter
Repeat the following steps to the number of waves you want divided by 2 .
- Draw the first line with the angle increments with positive values.
- Draw the second line with the angle increments with negative values.
Test Code
package main
import (
"github.com/disintegration/imaging"
"image/color"
"math"
)
func main() {
img := imaging.New(500, 300, color.White)
lengthOfLine := 100
numberOfWaves := 15
angle := 40
x0 := 10
y0 := 150
// using increments of 1
var x, y float64
angleInRadians := float64(angle) * (math.Pi / 180)
x = float64(x0)
y = float64(y0)
for j := 1; j <= numberOfWaves; j++ {
outOfMod := math.Mod(float64(j), 2.0)
outOfModInt := int(math.Floor(outOfMod))
if outOfModInt == 0 {
for i := 1; i < lengthOfLine; i++ {
x1 := x + (math.Cos(angleInRadians) * 1)
y1 := y + (math.Sin(angleInRadians) * 1)
img.Set(int(math.Round(x1)), int(math.Round(y1)), color.Black)
x = x1
y = y1
}
} else if outOfModInt == 1 {
for i := 1; i < lengthOfLine; i++ {
x1 := x + (math.Cos(-angleInRadians) * 1)
y1 := y + (math.Sin(-angleInRadians) * 1)
img.Set(int(math.Round(x1)), int(math.Round(y1)), color.Black)
x = x1
y = y1
}
}
}
err := imaging.Save(img, "out_swaves.png")
if err != nil {
panic(err)
}
}