Curly Waves - Maths317
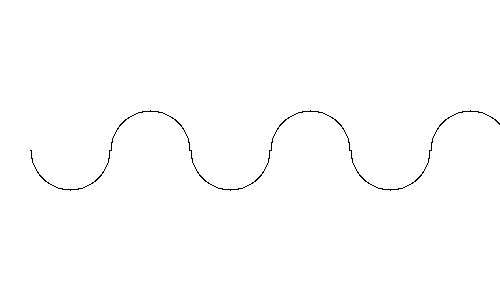
We would be using the line formula in the circles chapter
Repeat the following steps to the number of waves you want divided by 2 .
- Draw the first half of the circle with angles ranging from 90 to 270.
- Draw the second half of the circl with angles ranging from 270 to 450.
Test Code
package main
import (
"github.com/disintegration/imaging"
"image/color"
"math"
)
func main() {
width := 500
height := 300
img := imaging.New(width, height, color.White)
radius := 40
x0 := 70
y0 := 150
numberOfWaves := 15
for j := 1; j <= numberOfWaves; j++ {
outOfMod := math.Mod(float64(j), 2.0)
outOfModInt := int(math.Floor(outOfMod))
if outOfModInt == 0 {
for angle := 90; angle <= 270; angle++ {
angleInRadians := float64(angle) * (math.Pi / 180)
x := float64(radius) * math.Sin(angleInRadians)
y := float64(radius) * math.Cos(angleInRadians)
img.Set(x0 + int(x), y0 + int(y), color.Black)
}
x0 = x0 + (radius * 2)
} else if outOfModInt == 1 {
for angle := 270; angle <= 450; angle++ {
angleInRadians := float64(angle) * (math.Pi / 180)
x := float64(radius) * math.Sin(angleInRadians)
y := float64(radius) * math.Cos(angleInRadians)
img.Set(x0 + int(x), y0 + int(y), color.Black)
}
x0 = x0 + (radius * 2)
}
}
err := imaging.Save(img, "curly_waves.png")
if err != nil {
panic(err)
}
}